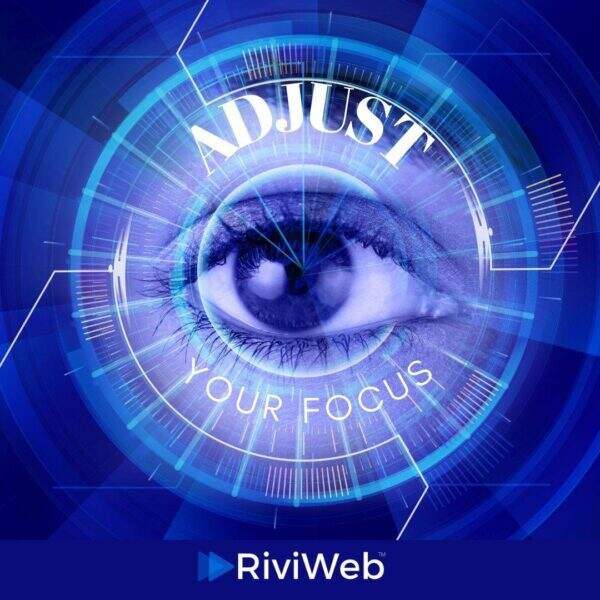
Coding in Web Development
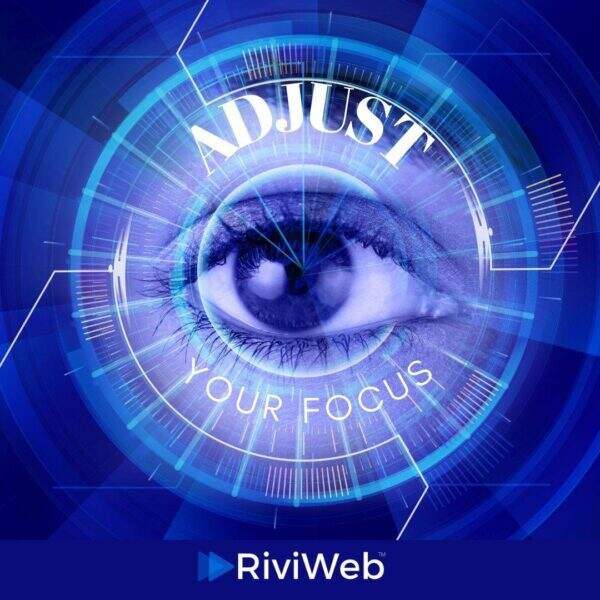
Why Posting on Platforms is Not a “Rinse and Repeat” Action
Understanding PHP and its Role in WordPress Development
PHP, or Hypertext Preprocessor, is a server-side scripting language that plays an essential role in the development of web applications, particularly dynamic websites. It is widely known for its use in web development, especially in creating content management systems (CMS), one of the most famous being WordPress. This blog post will explore what PHP is, how it works within WordPress, best practices for using PHP in WordPress development, the importance of updating PHP versions, and why WordPress developers should be aware of deprecated features.
What is PHP?
PHP is a scripting language that is primarily used for web development. PHP was created by Rasmus Lerdorf in 1993, PHP evolved from a set of Common Gateway Interface (CGI) scripts to a full-fledged programming language designed for building dynamic and interactive websites. Unlike static HTML, which is used to display fixed content, PHP allows developers to create dynamic content by interacting with databases, managing session information, handling form submissions, and much more.
PHP operates on the server side, meaning that the code is executed on the web server before the results are sent to the user’s browser. This makes it incredibly powerful for building websites that require frequent updates or involve complex data manipulations. A PHP file typically has a .php extension and is interpreted by a PHP processor on the web server.
One of PHP’s strengths lies in its ability to integrate easily with relational database management systems like MySQL, which is crucial for storing and retrieving data such as user profiles, posts, comments, etc., in the context of a CMS like WordPress.
PHP and WordPress
The Importance of PHP in WordPress
WordPress is the most popular content management system (CMS) in the world, powering over 40% of websites on the internet. WordPress is built using PHP, and the majority of its functionality, including themes, plugins, and core features, relies on PHP to function properly.
WordPress uses PHP to dynamically generate web pages. When you visit a WordPress site, the PHP code interacts with the database to retrieve information, such as blog posts or pages, and formats that data according to the theme and templates specified. The server executes the PHP code and returns the resulting HTML, CSS, and JavaScript to the user's browser for rendering. PHP is also used to create custom functionalities and extend the platform with plugins and themes.
How WordPress Uses PHP
Themes and Templates: WordPress themes are composed of PHP files that manage the structure of the website. Template files within a theme define how individual elements like the header, footer, and content are displayed. PHP functions within the templates retrieve data, such as post content, and format it for display.
Plugins: WordPress plugins are also built using PHP. Plugins extend the functionality of WordPress by adding new features. Examples include contact forms, SEO tools, custom post types, and e-commerce systems. Each plugin is a collection of PHP scripts that interact with WordPress core, themes, and the database to provide these additional functionalities.
Admin Dashboard: The WordPress admin panel, where site owners and administrators manage their content, themes, plugins, and settings, is powered by PHP. It enables users to create posts, upload media, moderate comments, and configure site settings.
Why PHP is Essential for WordPress
PHP provides WordPress with flexibility and speed. Since WordPress is dynamic, it constantly interacts with a database to retrieve and update content. PHP enables this interaction and ensures that content is served to users efficiently and quickly. The use of PHP makes it easier for developers to customize WordPress and create complex functionalities without needing to manually adjust HTML files or static pages.
Best Practices for PHP in WordPress Development
As WordPress is a constantly evolving platform, developers follow certain best practices when writing PHP code to ensure security, performance, and maintainability. Below are some essential practices that WordPress developers should follow when working with PHP.
- Use WordPress Built-in Functions and APIs
WordPress provides a rich set of functions and APIs that simplify common tasks such as database queries, content retrieval, and user authentication. Developers should always prefer using these built-in functions, over writing custom PHP code from scratch. For example, instead of directly querying the database using custom SQL, it’s best to use the WP_Query class or functions like get_posts() or get_user_meta().
Not only does this ensure that your code is optimized and efficient, but it also improves compatibility with future versions of WordPress.
- Follow WordPress Coding Standards
WordPress has established a set of coding standards for PHP that developers are encouraged to follow. These standards help maintain consistency across the WordPress ecosystem, making it easier to collaborate on development projects and maintain code. Some of these standards include:
Proper indentation and spacing
Clear and descriptive naming conventions for functions and variables
Use of comments for documenting code
Consistent use of the WordPress API for common tasks
By adhering to these coding standards, developers ensure that their code is readable and understandable by other developers working on the same project.
- Security Considerations
PHP code in WordPress often interacts with user inputs, such as form submissions or URL parameters. Developers must ensure that this data is sanitized and validated to prevent malicious attacks, such as SQL injection or cross-site scripting (XSS). WordPress provides several functions, such as sanitize_text_field() and esc_html(), which help secure user data.
Additionally, developers should avoid using functions like eval() or exec() that can lead to security vulnerabilities. Following security best practices is essential to protect both the WordPress site and its users.
- Optimize for Performance
As WordPress sites can have thousands of users visiting at once, performance is a key concern. Developers should optimize PHP code for speed and efficiency. Some best practices include:
Caching database queries when possible using WordPress’ Transients API
Using WP_Query effectively to limit the number of database queries
Minimizing the use of external resources
Using asynchronous loading for non-critical resources like JavaScript or CSS files
- Error Handling and Debugging
In a production environment, it is crucial to handle errors gracefully. WordPress provides a debugging tool called WP_DEBUG which helps developers identify issues in PHP code. Enabling error logging can also help in tracking down issues that affect site performance or cause crashes.
When working with plugins or themes, it is essential to test for potential errors before releasing code to ensure the site remains functional.
Why WordPress Should Use the Latest Version of PHP
PHP is constantly evolving, and each new version of PHP brings improvements in speed, security, and functionality. WordPress sites should use the latest stable version of PHP, as it offers several benefits:
- Improved Performance
Newer PHP versions typically have performance enhancements that make code execution faster. PHP 7 and later, for example, introduced major performance improvements that can significantly speed up the loading time of a WordPress site. As WordPress is built on PHP, updating to the latest PHP version can improve the overall site performance.
- Security Fixes
PHP releases regular security updates to patch vulnerabilities that could be exploited by attackers. Using an outdated version of PHP means your website is potentially exposed to security risks that can be easily exploited. Keeping PHP updated ensures that your site benefits from the latest security patches.
- Better Features and Functionality
New versions of PHP introduce new features and functionality that developers can leverage to enhance WordPress sites. Features like type declarations, improved error handling, and new built-in functions make it easier to write clean, maintainable, and efficient code.
- Compatibility with Plugins and Themes
Many modern WordPress plugins and themes require a more recent version of PHP. By keeping your PHP version updated, you ensure that you can take full advantage of the latest features and avoid compatibility issues with new plugins or themes that may not work on older PHP versions.
Why PHP Versions May Need to Be Changed
There are several reasons why you might need to change your PHP version in WordPress:
- Plugin Conflicts
WordPress plugins are created by different developers, and not all plugins are compatible with every version of PHP. Some plugins may require a specific PHP version to function properly. If a plugin is causing issues or errors, it may be incompatible with your current PHP version. In such cases, switching to a different PHP version may resolve the issue.
- Deprecated Features
PHP is an evolving language and older versions of PHP eventually phase out certain functions, features, or behaviors. This process is called "deprecation." Deprecated functions are features that are no longer recommended for use and may be removed entirely in future versions of PHP. When a feature is deprecated, it is still available but will trigger a warning in the code, signaling that it should no longer be used.
It’s important to note that deprecated functions do not stop your code from running, but relying on deprecated features can lead to future compatibility issues as PHP evolves.
- End of Support for Older Versions
PHP versions have a lifecycle, and older versions eventually reach their end of life (EOL). When PHP reaches its EOL, it no longer receives security updates or bug fixes. Running a WordPress site on an unsupported version of PHP puts the site at risk of being compromised.
What Does "Deprecated" Mean in PHP?
In PHP, "deprecated" refers to features, functions, or practices that are no longer recommended for use and are likely to be removed in future versions of PHP. A deprecated feature still works in the current version of PHP but generates a warning indicating that it will be removed in future releases. Developers are encouraged to stop using deprecated features and migrate to newer alternatives.
For example, in PHP 7, functions like mysql_connect() were deprecated in favor of mysqli_connect() and PDO, which are more secure and flexible options for interacting with a MySQL database.
Deprecation warnings alert developers to potential issues in their code that could break functionality when upgrading to future versions of PHP. Therefore, it’s essential to keep track of deprecated features and update your code to avoid compatibility problems in the future.
Conclusion
PHP is an essential part of WordPress development, providing the flexibility and functionality required to build dynamic websites and content management systems. By following best practices and staying up to date with the latest PHP versions, developers can ensure their WordPress sites are secure, fast, and compatible with modern features. Understanding the concept of deprecated features and the potential for conflicts with plugins also allows developers to maintain long-term site stability and performance. In today’s fast-paced digital landscape, using the latest version of PHP is crucial to maintaining a high-quality, secure WordPress site.